Lesson 7. How to get data via the API
In this lesson, you will learn:
In this lesson, you will learn about the API structure and look at some examples of getting data.
Let's remember how objects are linked in the Yandex Direct API (see Lesson 1):
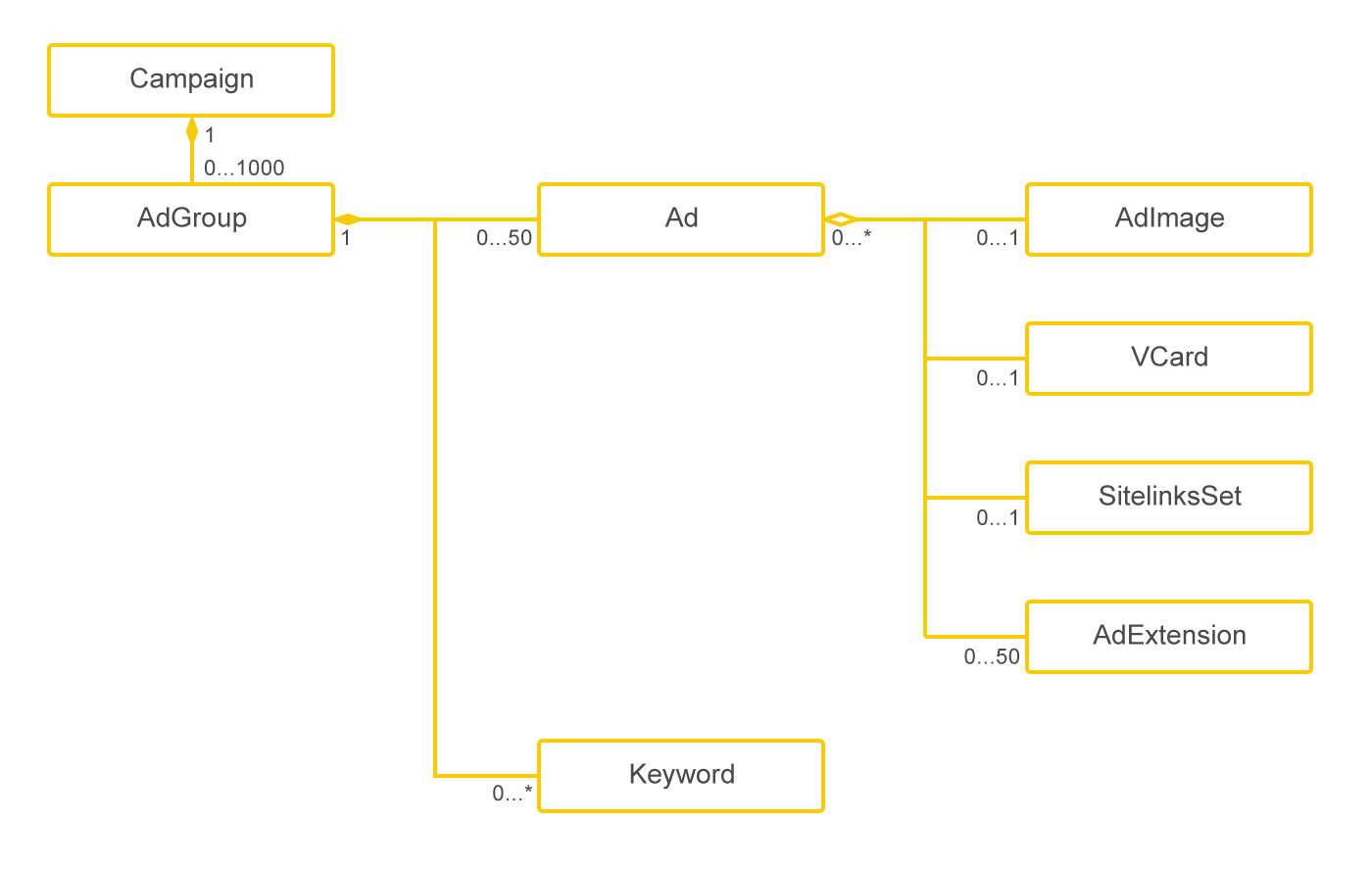
The API provides a service for each object class: Campaigns, for campaign management; Groups, for ad group management; Ads, for ad management, etc. For the full list of services please refer to the documentation.
The logic of working with the API objects is unified, with all the service methods assigned standard names. A service typically includes the following methods:
- add — Adding objects.
- update — Changing object parameters.
- delete — Deleting objects.
- get — Getting objects.
A service can also have other methods. For example, the Campaigns service provides the following methods:
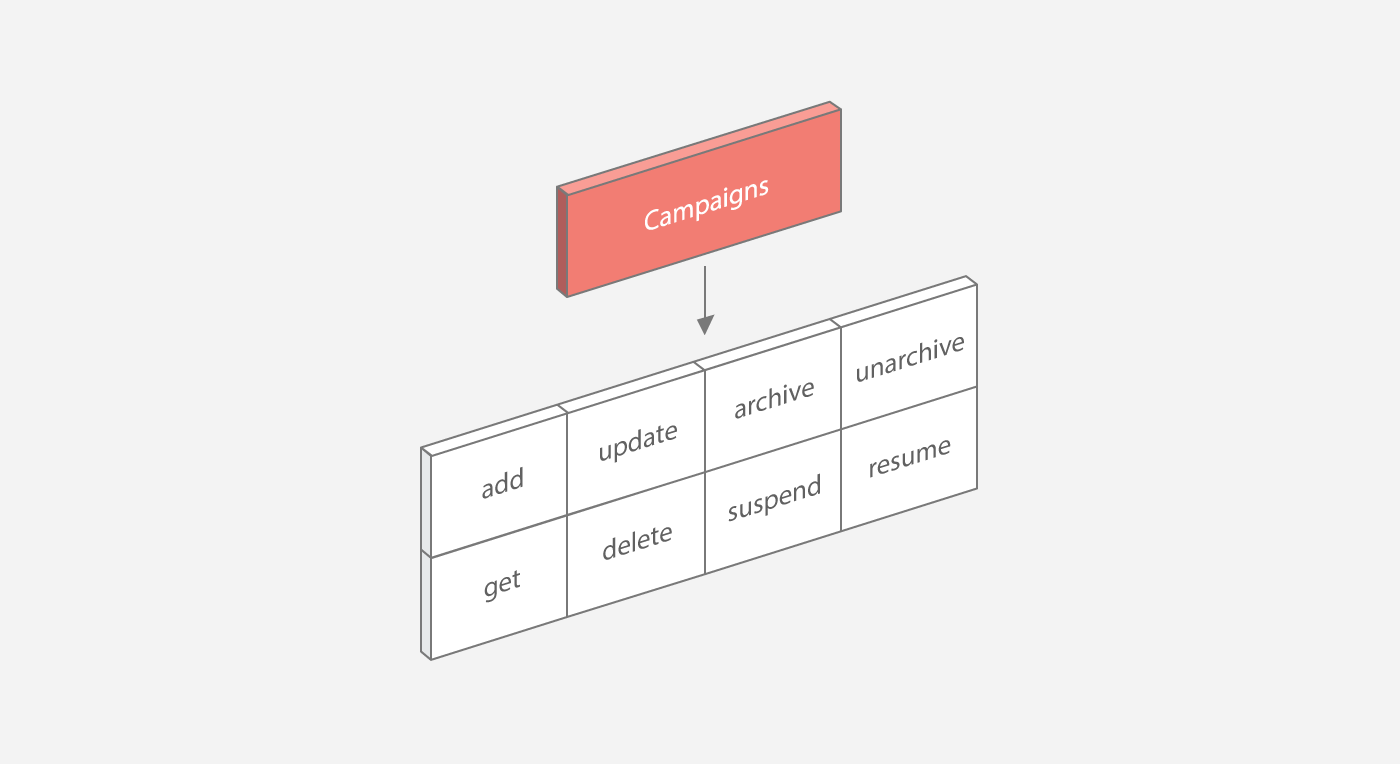
How to get objects
Let's look how you can get ad campaign parameters using the get method.
The get method is designed so that you can request only the data you need. For example, for campaigns you can get their IDs and names only, rather than all their parameters. List the names of the parameters you need in the FieldNames input parameter.
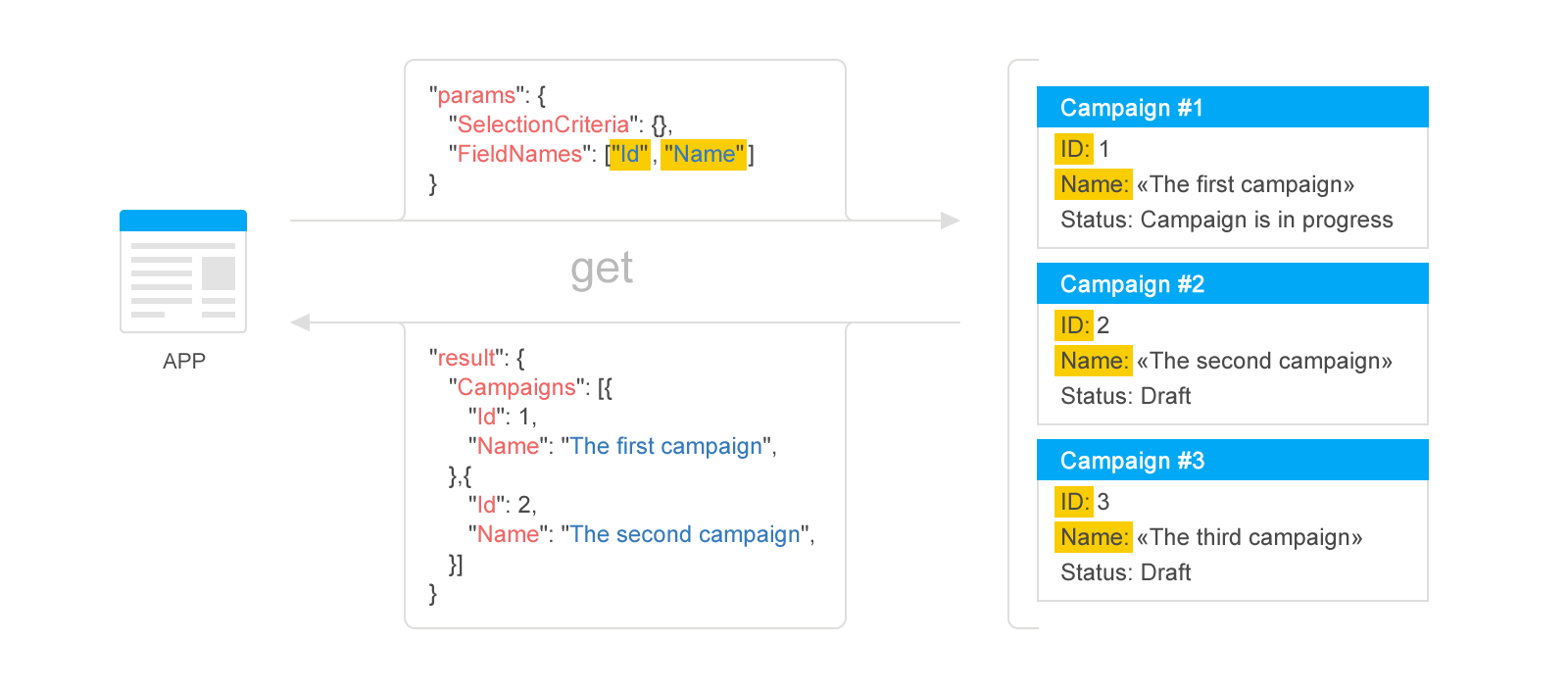
Use the SelectionCriteria input structure to set criteria for selecting objects. If there are several selection criteria, the server searches for objects fitting all the criteria at once. Some services allow you to specify an empty SelectionCriteria structure: in this case, all objects are returned.
Sandbox practice
Let's remember the query example from the previous lesson. In that example, we got an entire list of campaigns by specifying an empty SelectionCriteria structure, getting only an ID and name for each campaign by specifying relevant parameters in FieldNames.
- cURL
curl -k -H "Authorization: Bearer TOKEN" -d '{"method":"get","params":{"SelectionCriteria":{},"FieldNames":["Id","Name"]}}' https://api-sandbox.direct.yandex.com/json/v5/campaigns
- cURL for Windows
curl -k -H "Authorization: Bearer TOKEN" -d "{\"method\":\"get\",\"params\":{\"SelectionCriteria\":{},\"FieldNames\":[\"Id\",\"Name\"]}}" https://api-sandbox.direct.yandex.com/json/v5/campaigns
- Request
{ "method": "get", "params": { "SelectionCriteria": {}, "FieldNames": ["Id", "Name"] } }
- Response
{ "result": { "Campaigns": [{ "Name": "Test API Sandbox campaign 1", "Id": 1234567 }, { "Name": "Test API Sandbox campaign 2", "Id": 1234578 }, { "Name": "Test API Sandbox campaign 3", "Id": 1234589 }] } }
Some campaign parameters (such as name and ID) are shared across all campaign types, while some of them (such as display strategies) depend on the campaign type. In the next sample, we only get campaigns of the “Text & Image Ads” type. To get display strategies for those campaigns, specify the relevant parameter name in the TextCampaignFieldNames input parameter.
- cURL
curl -k -H "Authorization: Bearer TOKEN" -d '{"method":"get","params":{"SelectionCriteria":{ "Types": ["TEXT_CAMPAIGN"]},"FieldNames":["Id","Name"],"TextCampaignFieldNames":["BiddingStrategy"]}}' https://api-sandbox.direct.yandex.com/json/v5/campaigns
- cURL for Windows
curl -k -H "Authorization: Bearer TOKEN" -d "{\"method\":\"get\",\"params\":{\"SelectionCriteria\":{ \"Types\": [\"TEXT_CAMPAIGN\"]},\"FieldNames\":[\"Id\",\"Name\"],\"TextCampaignFieldNames\":[\"BiddingStrategy\"]}}" https://api-sandbox.direct.yandex.com/json/v5/campaigns
- Request
{ "method": "get", "params": { "SelectionCriteria": { "Types": ["TEXT_CAMPAIGN"] }, "FieldNames": ["Id", "Name"], "TextCampaignFieldNames": ["BiddingStrategy"] } }
- Response
{ "result": { "Campaigns": [{ "Id": 1234567, "Name": "Test API Sandbox campaign 1", "TextCampaign": { "BiddingStrategy": { "Search": { "BiddingStrategyType": "HIGHEST_POSITION" }, "Network": { "BiddingStrategyType":"NETWORK_DEFAULT", "NetworkDefault": { "LimitPercent": 100 } } } } }] } }
- Paginated selection
- If you are working with large data arrays, all the objects might not fit in a single request response, since the get method returns a maximum of 10,000 objects. In this case, the get method returns the LimitedBy parameter with a sequential number of the last object returned. If the response contains this parameter, it indicates that you haven't got all the objects yet.
Task
We have prepared some samples for other services as well. Try to reproduce these requests in Sandbox.
- AdGroups service
-
Get a list of ad groups for a campaign.
cURLcurl -k -H "Authorization: Bearer TOKEN" -d '{"method":"get","params":{"SelectionCriteria":{"CampaignIds":[CAMPAIGN_IDENTIFIER]},"FieldNames":["Id","Name","Status","Type"]}}' https://api-sandbox.direct.yandex.com/json/v5/adgroups
cURL for Windowscurl-k-H "Authorization: Bearer token" - d "{\"method\":\"get\",\"params\":{\"SelectionCriteria\":{\"CampaignIds\":[CAMPAIGN_IDENTIFIER]},\"FieldNames\":[\"Id\",\"Name\",\"Status\",\"Type\"]}}" https://api-sandbox.direct.yandex.com/json/v5/adgroups
Request{ "method": "get", "params": { "SelectionCriteria": { "CampaignIds": [CAMPAIGN_ID] }, "FieldNames": ["Id", "Name", "Status", "Type"] } }
Response{ "result": { "AdGroups": [{ "Status": "ACCEPTED", "Name": "Group #1296502", "Id": 1296502, "Type": "TEXT_AD_GROUP" }, { "Name": "Group #1296517", "Status": "DRAFT", "Id": 1296517, "Type": "TEXT_AD_GROUP" }] } }
- Ads service
-
Get the list of ads in an ad group.
cURLcurl -k -H "Authorization: Bearer TOKEN" -d '{"method":"get","params":{"SelectionCriteria":{"AdGroupIds":[AD_GROUP_IDENTIFIER]},"FieldNames":["Id","State","Status","Type"]}}' https://api-sandbox.direct.yandex.com/json/v5/ads
cURL for Windowscurl -k -H "Authorization: Bearer TOKEN" -d "{\"method\":\"get\",\"params\":{\"SelectionCriteria\":{\"AdGroupIds\":[AD_GROUP_IDENTIFIER]},\"FieldNames\":[\"Id\",\"State\",\"Status\",\"Type\"]}}" https://api-sandbox.direct.yandex.com/json/v5/ads
Request{ "method": "get", "params": { "SelectionCriteria": { "AdGroupIds": [GROUP_ID] }, "FieldNames": ["Id", "State", "Status", "Type"] } }
Response{ "result": { "Ads": [{ "State": "ON", "Id": 1381459, "Status": "ACCEPTED", "Type": "TEXT_AD" }, { "Type": "TEXT_AD", "Status": "DRAFT", "Id": 1381470, "State": "OFF" }] } }
Get parameters of a single ad.
cURLcurl -k -H "Authorization: Bearer TOKEN" -d '{"method":"get","params":{"SelectionCriteria":{"Ids":[AD_IDENTIFIER]},"FieldNames":["Id"],"TextAdFieldNames":["Text","Title","Href","VCardId"]}}' https://api-sandbox.direct.yandex.com/json/v5/ads
cURL for Windowscurl -k -H "Authorization: Bearer TOKEN" -d "{\"method\":\"get\",\"params\":{\"SelectionCriteria\":{\"Ids\":[AD_IDENTIFIER]},\"FieldNames\":[\"Id\"],\"TextAdFieldNames\":[\"Text\",\"Title\",\"Href\",\"VCardId\"]}}" https://api-sandbox.direct.yandex.com/json/v5/ads
Request{ "method": "get", "params": { "SelectionCriteria": { "Ids": [1381459] }, "FieldNames": ["Id"], "TextAdFieldNames": ["Text", "Title", "Href", "VCardId"] } }
Response{ "result": { "Ads": [{ "Id": 1381459, "TextAd": { "Title": "Test sandbox banner 5", "Href": "http://www.yandex.ru", "VCardId": 171518, "Text": "Test sandbox banner 5 text" } }] } }
- Keywords service
-
Get keywords for an ad group.
cURLcurl -k -H "Authorization: Bearer TOKEN" -d '{"method":"get","params":{"SelectionCriteria":{"AdGroupIds":[AD_GROUP_IDENTIFIER]},"FieldNames":["Id","Keyword","Bid","State","Status"]}}' https://api-sandbox.direct.yandex.com/json/v5/keywords
cURL for Windowscurl -k -H "Authorization: Bearer TOKEN" -d "{\"method\":\"get\",\"params\":{\"SelectionCriteria\":{\"AdGroupIds\":[AD_GROUP_IDENTIFIER]},\"FieldNames\":[\"Id\",\"Keyword\",\"Bid\",\"State\",\"Status\"]}}" https://api-sandbox.direct.yandex.com/json/v5/keywords
Request{ "method": "get", "params": { "SelectionCriteria": { "AdGroupIds": [1296506] }, "FieldNames": ["Id", "Keyword", "Bid", "State", "Status"] } }
Response{ "result": { "Keywords": [{ "State": "ON", "Keyword": "test keyword 5.1", "Status": "ACCEPTED", "Bid": 7700000, "Id": 3458327 }, { "Keyword": "New keyword", "Id": 3458351, "Bid": 400000, "Status": "ACCEPTED", "State": "ON" }] } }
What's next
So, you have learned how to use the get method. In the next lesson, you will learn about the methods that change the data.
Useful links
Documentation: How the "get" method works
Questions
- What is the purpose of the Yandex Direct Campaigns API service?
False.
False.
False.
True.
- Which method of the Campaigns service can you use to get data?
False.
False.
True.
False.
- Why would you use paginated selection?
True.
False.
False.